PHP8.3 is due to release on the 23rd November, 2023. Let's have a look over a couple of the new features that are to come!
New json_validate Function
Previously if you wanted to validate JSON was valid, you would have to call json_decode
on it and check whether the return result is null, or by adding the JSON_THROW_ON_ERROR
flag to catch a thrown exception.
$decodedJson = json_decode($jsonString, true);
if (null === $decodedJson) {
$error = json_last_error_msg();
// Rest of the error handling...
}
By using json_validate
, you no longer need to decode the JSON in order to check its validity. As pointed out in the RFC, this won't impact 99% of users since usually you're looking to decode JSON over validating it.
So why is it needed?
In the event of meeting use cases where users need to validate JSON, ie: from user input. This meant rolling out your own validator (complex and time-consuming) or using the less-efficient json_decode
. Since PHP had JSON validation internally, they're now exposing it for everyone to use.
Using json_validate
is for the most part similar to json_decode
and accepts similar parameters, apart from the $associative parameter since there's no data to return.
$jsonValid = json_validate($jsonString);
if (! $jsonValid) {
$error = json_last_error_msg();
// Rest of the error handling...
}
New Type Class Constants
PHP has seen incremental improvements to its type definitions over the last couple of releases. PHP 8.3 continues this with the addition of class constant types.
What does this mean? Consider how we could already define types on properties like so:
class Foo {
public string $name = 'Bob';
}
We can now also extend types to constants.
class Foo {
public const string STATUS_IN_PROGRESS = 'In Progress';
}
And if we attempt to set a value that doesn't satisfy the type:
class Foo {
public const string STATUS_IN_PROGRESS = 1;
}
Then you should expect to receive a fatal error:
Fatal error: Cannot use int as value for class constant Test::CONST of type string
New Dynamic Class Constant and Enum Fetch
Sticking with the theme of constants. In PHP8.3 it will be possible to fetch a constant or enum via a variable name dynamically.
Let's go over an example. Prior to PHP8.2, to access a constant dynamically you would have to use the constant
function:
class Foo {
public const STATUS_IN_PROGRESS = 'In Progress';
}
$status = 'STATUS_IN_PROGRESS';
echo constant("Foo::$status") // echos out "In Progress"
Now in PHP8.3, you can use the new syntax to access a constant dynamically:
class Foo {
public const STATUS_IN_PROGRESS = 'In Progress';
}
$status = 'STATUS_IN_PROGRESS';
echo Foo::{$status}; // echos out "In Progress"
The same goes for enums too:
enum Status: string {
case InProgress = 'In Progress';
}
$status = 'InProgress';
echo Status::{$status}->value; // echos out "In Progress"
New mb_str_pad
mb_str_pad is the multibyte counterpart of str_pad. If you are not familiar with str_pad, it allows you to pad a string to a desired length with a choice of direction and characters to pad with.
Take the following example where str_pad
is used to pad out a stockId value.
$stockId = '100';
str_pad($stockId, 8, '0', STR_PAD_LEFT); // outputs: 00000100
So this is all good, until we need to work with multibyte strings.
Take this example:
$name = 'ボッブ';
echo str_pad($name, 8, '_', STR_PAD_LEFT); // outputs: ボッブ
Nothing changes. This is because ボッブ is actually 9 bytes long, and we're padding to the count of 8. You can see this if you do strlen on the value of $name, instead of 3 you'll see 9. Changing to mb_strlen
will output 3, as you might expect.
Finally, to get the result you might expect. Let's change over to mb_string_pad
for the above example.
$name = 'ボッブ';
echo mb_str_pad($name, 8, '_', STR_PAD_LEFT); // outputs: _____ボッブ%
New Randomizer Extension Methods
PHP8.2 introduced a new Randomizer extension that allows for an object-oriented way of retrieving randomised integers or bytes.
PHP8.3 extends the methods available by adding randomisation for floats: Randomizer::getFloat()
, and Randomizer::nextFloat()
.
Additionally, a new Randomizer::getBytesFromString()
has been added. Handy for generating a string of random letters from a given string.
$random = new Random\Randomizer();
$letters = "abcdef";
$random->getBytesFromString($letters, 5); // "cabef"
When is PHP8.3's Release Date?
PHP8.3 is scheduled to be released on 23rd November, 2023.
Can I Try PHP8.3 Early?
If you're on macOS, Taylor Otwell, (the creator of Laravel) sponsored the development of Herd. An awesome free tool for helping you work with multiple versions of PHP.
With Herd, you can install multiple versions of PHP all at once and individually set which version to use for specific projects. But the best part is it removes the frustration of fighting package managers or waiting for stable releases.
You can go ahead and install PHP8.3 right away!
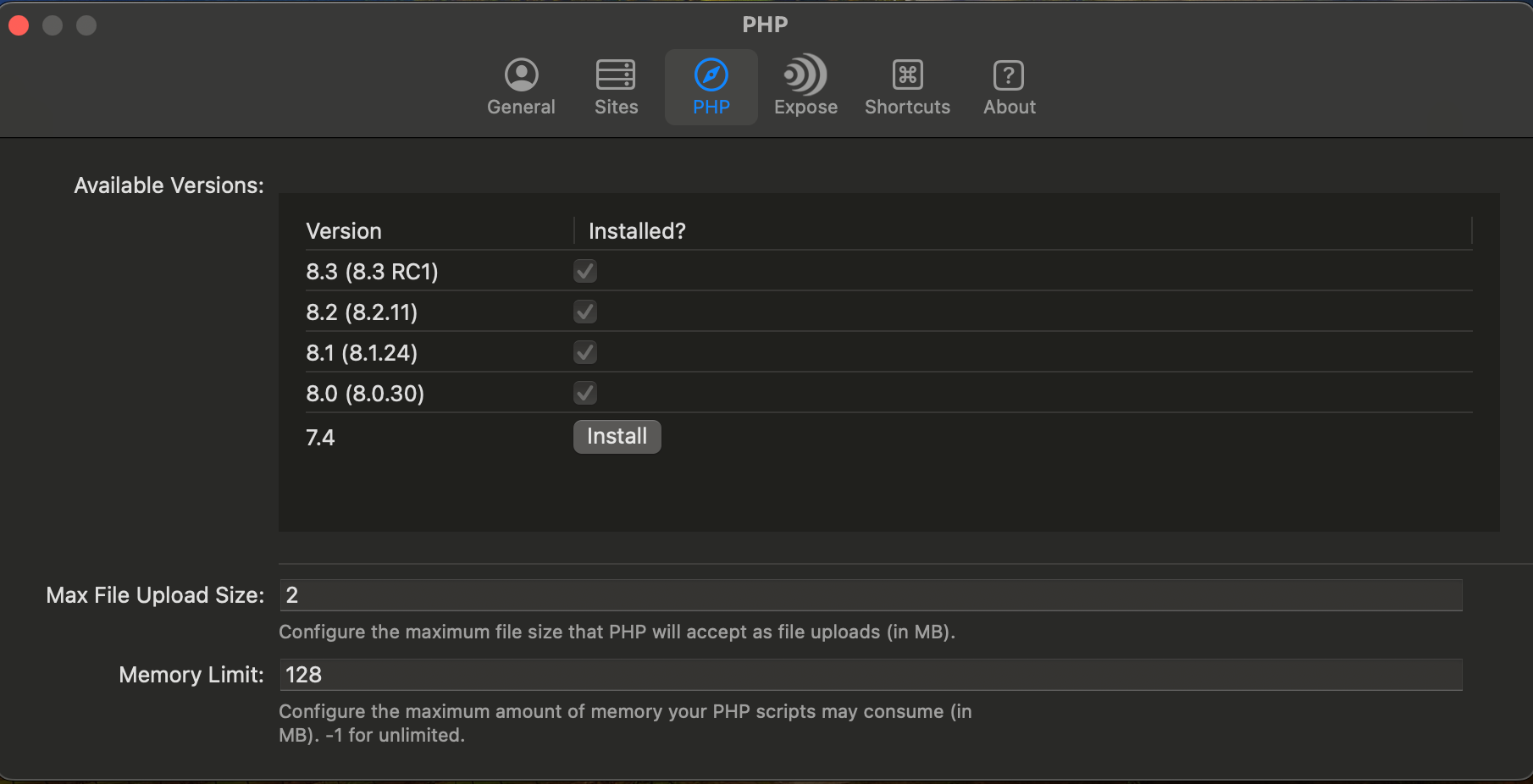