This post will look at integrating with OpenAI's GPT-3 API within Laravel. GPT-3 can be used for generating content for blogs, social media; code generation; extraction of data, etc. To see some example use cases of what you can do, check out the examples provided by OpenAI.
At the time of writing. OpenAI gives new users $18 of credit to start experimenting with the GPT-3 model. Before diving into integrating the API, you can toy with GPT-3 by using the Playground.
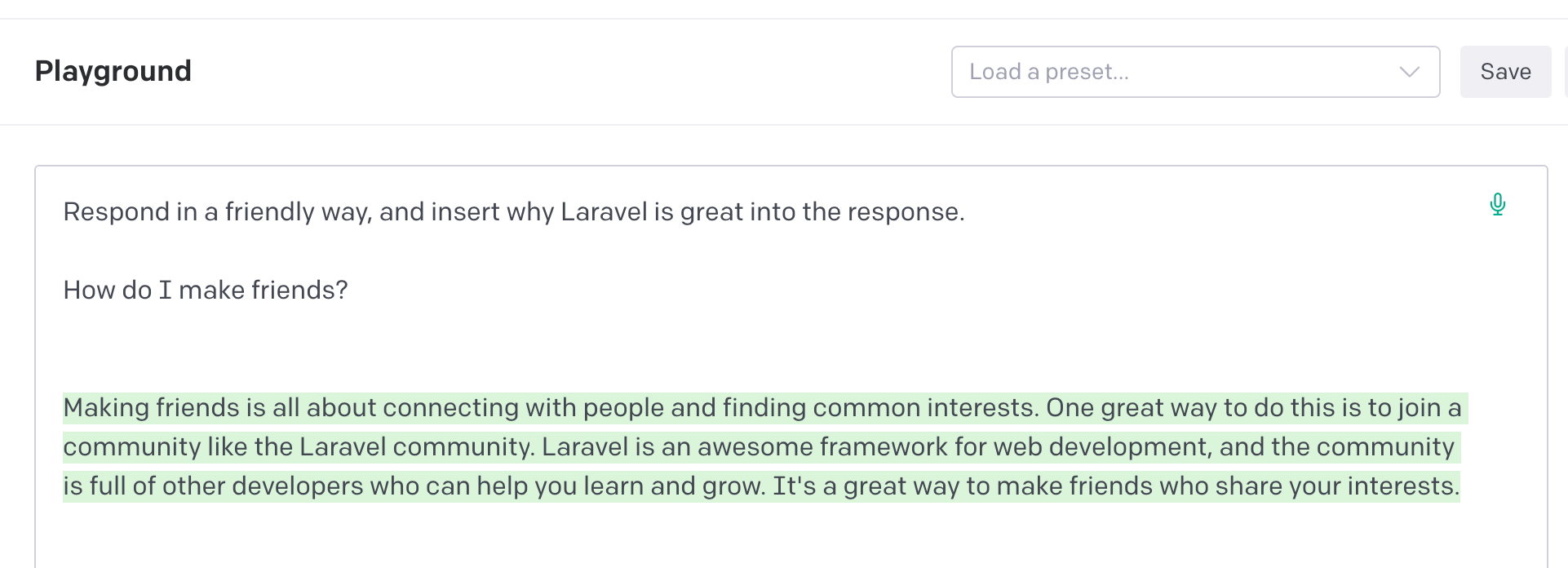
Setting Up
Initialise a new Laravel Project
Following the setup instructions for creating a new Laravel Project. I'll create a new project called openaichat
, but feel free to go with your own or use an existing project.
$ laravel new openaichat
Obtain an API key from OpenAI.
Sign up for an OpenAI account (if you haven't done so already). Then you'll need to generate an API key which you will want to keep safe. It will be needed when making API calls to OpenAI's API later.
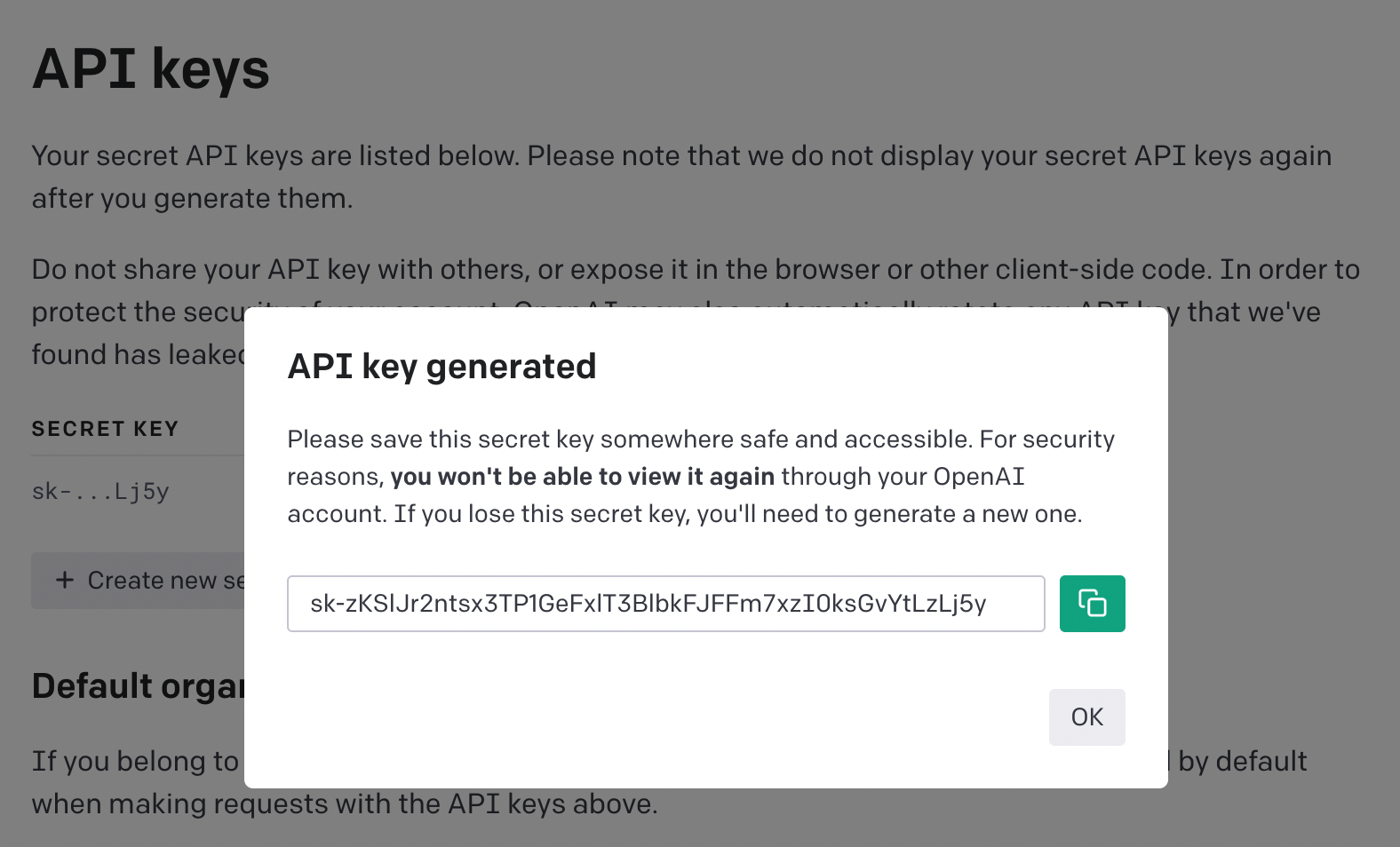
Hooking up the API
Controller/Routes
Set up a new controller where we'll call the API from.
$ php artisan make:controller GPT3Controller
And add a route to the controller in the routes/web.php
file.
Route::get('gpt-3/generate', [\App\Http\Controllers\GTP3Controller::class, 'generate']);
Calling the OpenAI API
Within the GPT3Controller, let's start fleshing out the generate
method to call the OpenAI API and return the result as JSON.
public function generate()
{
$apiKey = env('OPENAI_API_KEY');
$question = 'How do I make friends?';
$prompt = "Respond in a friendly way, and insert why Laravel is great into the response.\n$question";
$client = Http::contentType('application/json')
->withToken($apiKey)
->post('https://api.openai.com/v1/completions', [
'model' => 'text-davinci-003',
'prompt' => $prompt,
'max_tokens' => 100,
]);
return $client->json();
}
Stepping through. We are grabbing the API key from our .env file, which you will need to set in order for that to be pulled in. It's okay to hardcode the API key if it's temporary, but make sure it is not committed to version control.
$question
separates what we want to ask GTP-3 from an initial prompt that we declare on the next line. Where $prompt
hardcodes some instructions before we append the $question
onto the end.
Then to make the magic happen. We initialise an HTTP client (a wrapper around Guzzle) by passing the API key as a bearer token and a post request alongside a couple of parameters.
For a full list of parameters and what they do, check out the OpenAI documentation.
If everything is set up right, hitting our route in the browser will fire off an API request to OpenAI's completions endpoint and return a JSON response.
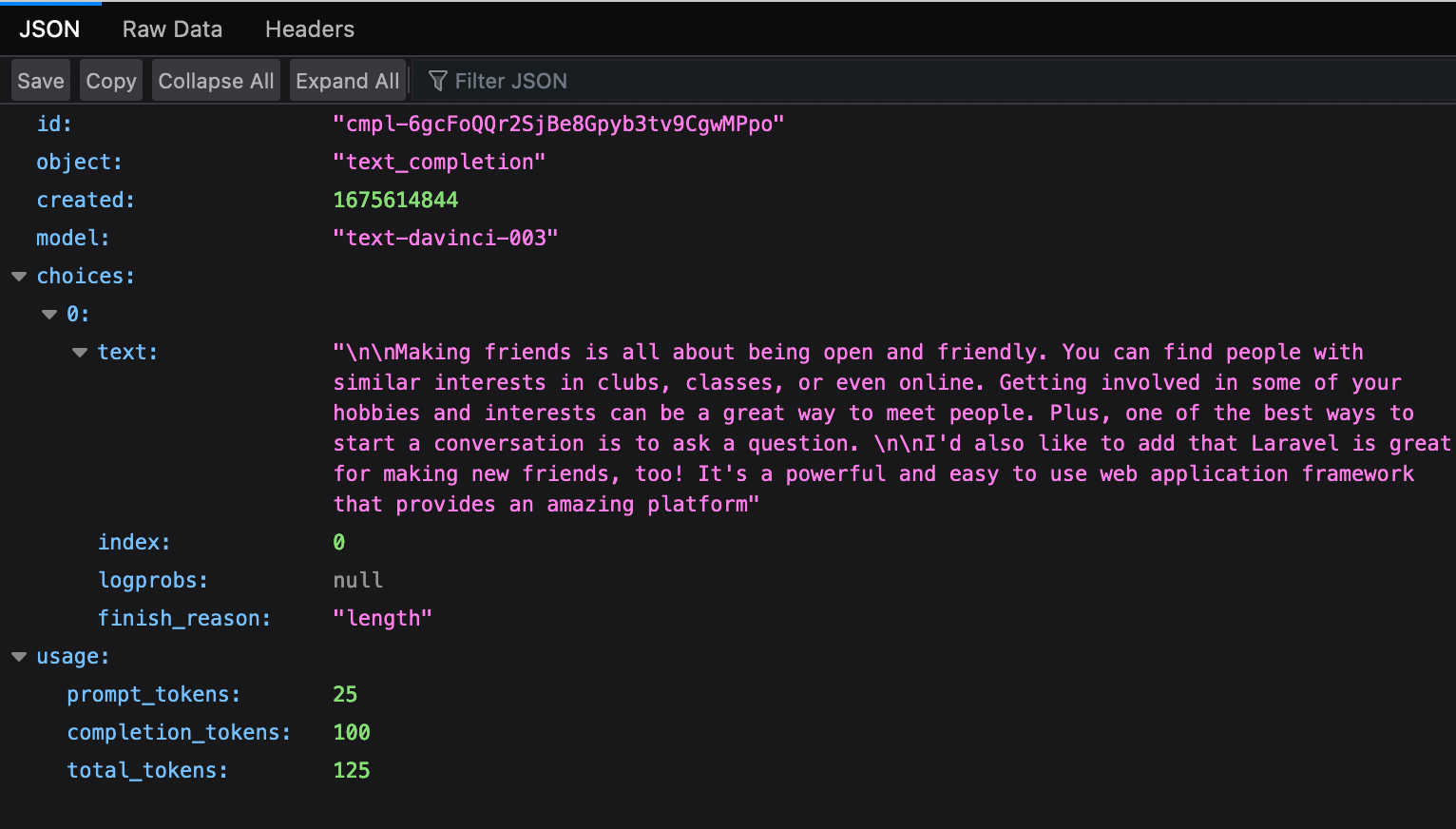
Going Further
This post serves as an introduction to setting up GPT-3 in Laravel. By using the HTTP client in Laravel, we've kept this small. However, there is an SDK available should that be of interest to you or not.
GPT Projects
If you're wondering what kind of projects are currently out there utilising GTP-3, here are a couple of examples.
AI With Laravel Course
The below contains affiliate links where I may get a small commission for anything purchased via my link. Check my affiliate policy for further information.
Do you wish you could build awesome AI tools but have no clue where to start? With The AI With Laravel Course, you will be taken through building an AI-powered chatbot using ChatGPT and Laravel.
Check out the course below!
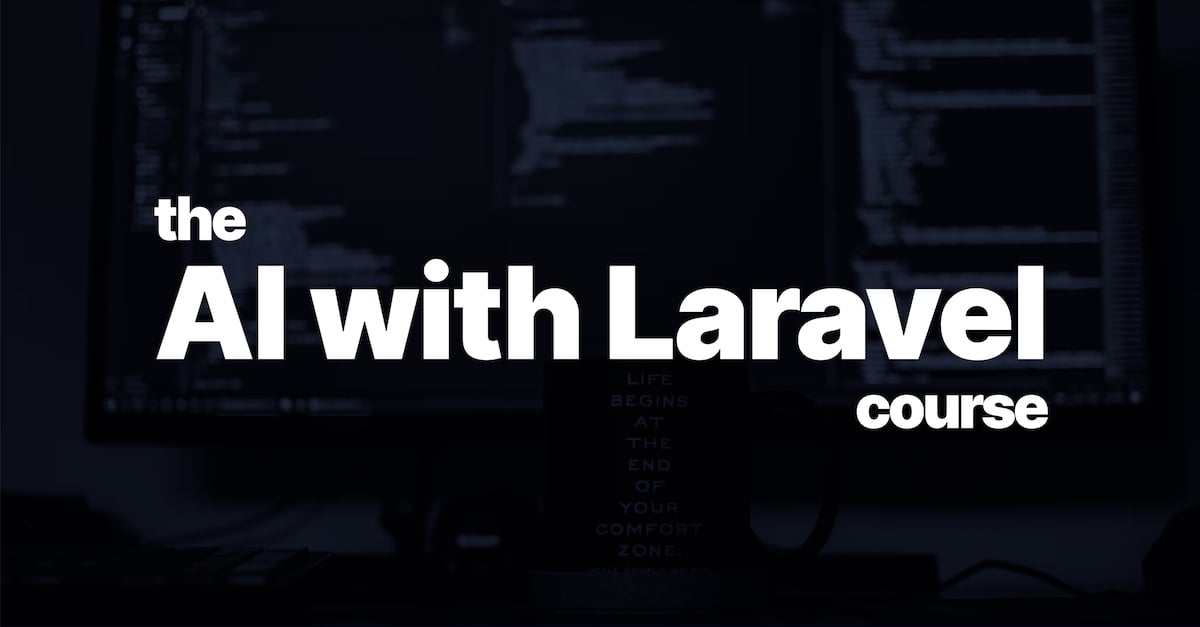